If you’ve used JavaScript much, you’ll probably know one of its very convenient features — the ability to assign functions to variables, send them as arguments, and return them as values. This lets you write code like this, where functions themselves return other functions:
1 | /* |
The problem with this, however, is that all the functions in the above example are anonymous — they aren’t named. Beyond making your code more obscure than it needs to be, this also makes debugging them a problem, because when you look at the browser’s call stack, you’re going to get something like this:
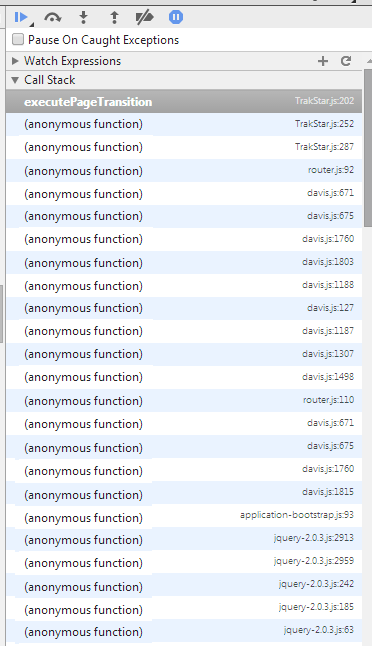
Yeah. Good luck with that.
However, you can remedy the issue quite easily &mdash because JavaScript lets you name your function variables:
1 | var myFunction = function myFunction(x) {...} |
…name your function values…
1 | compose(function someComposedFunction(x) {...} ) |
…and name returned functions…
1 | function lambda() { |
We can apply this syntax to our curry example. Let’s look again:
1 | /* |
Because the function variables are named, they’ll now be much easier to debug:
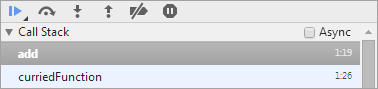
Naming your function variables makes debugging much easier, takes little effort, and arguably can make for better readability too.